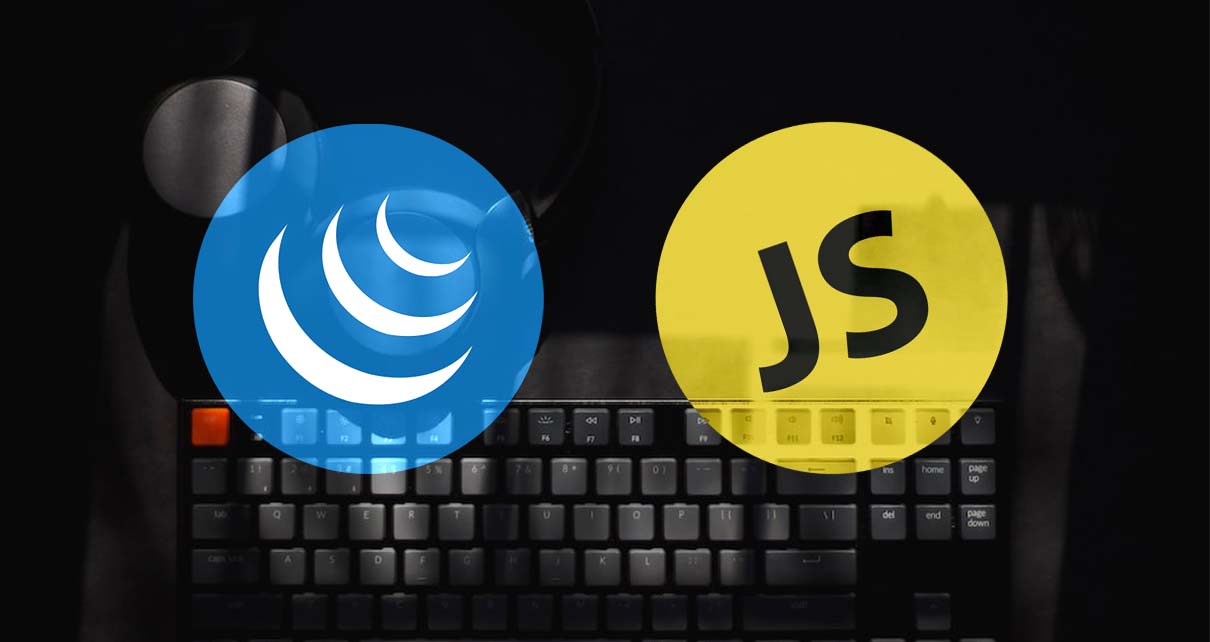
We offer two solutions. One with JavaScript and the other with JQuery. To personalize the template, we consider Bootstrap.
We offer two solutions. One with JavaScript and the other with JQuery. To personalize the template, we consider Bootstrap.
Below you can see how it works:
You can download the code from my repository @Github or copy/paste it from JSFiddle.
First we show the JQuery example and then the JavaScript solution.
1. JQuery
The code comprises two files. The template select-option-jquery.html
file reads:
<html>
<head>
<!-- bootstrap 5.2.3-->
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.3/dist/css/bootstrap.min.css" rel="stylesheet">
<!-- style sheet -->
<link href="style.css" rel="stylesheet">
</head>
<body>
<div class="container col-6 mt-4">
<!-- select option -->
<div>
<select class="form-select" id="list">
<option value="item1" selected>text 1</option>
<option value="item2">text 2</option>
<option value="item3">text 3</option>
</select>
</div>
<div class="p-2">
<div id="option-item1" class="option" style="display: block;">
Result 1 content
</div>
<div id="option-item2" class="option" >
Result 2 content
</div>
<div id="option-item3" class="option">
Result 3 content
</div>
</div>
</div>
<!-- bootstrap 5.2.3 -->
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.3/dist/js/bootstrap.bundle.min.js"></script>
<!-- jquery 3.6.3-->
<script src="https://code.jquery.com/jquery-3.6.3.min.js"></script>
<!-- jquery select option -->
<script>
$(document).ready(function() {
var $my_list = $( '#list' );
var $my_option = $( '.option' );
$my_list.on( 'change', function ( e ) {
$my_option.hide();
$( '#option-' + this.value ).show(); //option-item1, option-item2, or option-item3
});
})
</script>
</body>
</html>
The style sheet style.css
file reads:
.option {
display: none;
}
2. JavaScript
The code comprises one file. The template select-option-js.html
file reads:
<html>
<head>
<!-- bootstrap 5.2.3-->
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.3/dist/css/bootstrap.min.css" rel="stylesheet">
</head>
<body>
<div class="container col-6 mt-4">
<!-- select option -->
<div>
<select class="form-select" onchange="selectFunction(this)">
<option value="item1" selected>text 1</option>
<option value="item2" >text 2</option>
<option value="item3" >text 3</option>
</select>
</div>
<div class="p-2">
<div id="option-js-item1" class="option-js" style="display: block;">
Result 1 content
</div>
<div id="option-js-item2" class="option-js" style="display: none;">
Result 2 content
</div>
<div id="option-js-item3" class="option-js" style="display: none;">
Result 3 content
</div>
</div>
</div>
<!-- bootstrap 5.2.3 -->
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.3/dist/js/bootstrap.bundle.min.js"></script>
<!-- javascript select option -->
<script>
function selectFunction(selected){
var value = selected.value;
let option_item = document.getElementsByClassName('option-js')
for (var i = 0; i < option_item.length; i++ ) {
option_item[i].style.display = 'none';
}
document.getElementById('option-js-'+value).style.display = 'block';
}
</script>
</body>
</html>
Note: The Bootstrap files are not needed. They were included in the code to customize the template.
Views: 1 Github
Notifications
Receive the new articles in your email